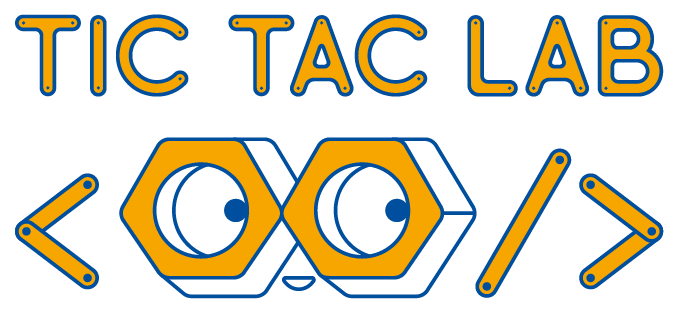
🗨️ Pour la version francaise, clique ici!
Before you start this challenge, make sure you have already done these:
Secondly, make sure you are highly motivated and eager to learn!
Also, make sure you are logged into your Repl.It account, and that you have your project from last time.
When you fall off platforms, you fall forever... That's not the point! So let's make sure we lose when we fall too low!
To see the X and Y coordinates of the player, we use
this.player.x;
this.player.y;
Try to log the coordinates in the console by writing this code in the update
function:
console.log(/* X coordinate */);
console.log(/* Y coordinate */);
Watch the numbers change when you move. You are logging the right coordinates!
To lose, we check if the Y coordinate is too high, and if it is, we change the Y and X coordinates so that our character moves towards the beginning of the game.
if(/* Y coordinate */ > /* Number you'd like */) {
/* X coordinate */ = /* Starting X */;
/* Y coordinate */ = /* Starting Y */;
}
You should now reappear at the beginning of the game when you fall off the map.
Let's add a victory object! Draw this lovely object in Pixel Art. You can use pixilart.com again.
Once drawn, add your new object to your project, as you did with your other drawings.
Now, load this new sprite (character).
this.load.image("coin", "coin.png");
Once your image is loaded into the project, we want it to appear somewhere. Again, easy!p>
this.coin = this.physics.add.sprite(500, 160, "coin");
You will of course have to change the X (500) and Y (160) coordinates in your game!
And now for the hard bit! Hang in there!
In the update
function, we'll check if you hit the victory coin. If you do, we'll stop the game, and display that you won!
To do something when you touch the coin, we use this code:
this.physics.add.overlap(this.player, this.coin, function(player, coin) {
// What we do when we touch the coin
}, null, this);
To make sure everything is working properly, console.log
the word "Touched". Remember that to log a word, you have to do
console.log(/* My word surrounded by quotation marks */);
It works? Great!
Now we'll make the coin disappear. Otherwise, you'll just keep winning forever, as you keep touching it!
coin.destroy();
Try it now: the coin should fade away when touched.
Now we add the text. To do this, we will first create the text:
let victoryText = this.add.text(0, 0, "VICTORY");
We have created the victory text, but we don't display it yet! To display it, you can do
Phaser.Display.Align.In.Center(victoryText, this.add.zone(400, 250, 400, 250));
This code looks rather complicated, doesn't it? Let's go through it section by section:
Phaser.Display.Align.In.Center
tells the program to display something number 1 in the middle of something number 2victoryText
is the number 1 something, which we want to display on the screenthis.add.zone(400, 250, 400, 250)
is something number 2, the zone in which we want to display our text.Excellent! But the text is a bit small, isn't it? How can we make it bigger?
Let's change the variable victoryText
to
let victoryText = this.add.text(0, 0, "VICTORY", {
fontSize: "50px",
});
Likewise, we can also add a color:
let victoryText = this.add.text(0, 0, "VICTORY", {
fontSize: "50px",
color: "#d53636",
});
You can of course choose your own font size and color!
Finally, we'd like to make sure that the game ends when we take the coin! We'll just pause the game, and never "un-pause" it
this.physics.pause();
Imagine that you want a world that is longer than your screen, and that you can move through it easily. The world would have to move with your character.
It's easy to do! In the create
function, add
this.cameras.main.startFollow(this.player);
This will make the camera follow your character everywhere. You can now create a very, very large world. Having said that, it would be better if the camera only followed our character in the horizontal direction (the x-axis).
To do this, add this line to the update
function:
this.cameras.main.setFollowOffset(0, this.player.y-250);
Small problem: now, when you win, the text is no longer in the middle 😓
To fix this, change the code where you create the text to this (just add .setScrollFactor(0)
)
let victoryText = this.add.text(0, 0, "VICTORY", {
color: "#d53636",
fontSize: "70px"
}).setScrollFactor(0);
VOILA! Now you can start building a much longer world than before. Create a nice obstacle game that we can try!